Vue的非父子组件通信
可以通过vuex和event bus来解决
Demo
我们要实现的效果是:
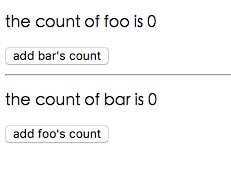
上下分别为foo组件和bar组件,他们之间是非父子关系,分别点击各自的button,另一个组件的count对应增加。
|
|
JavaScript的实现是:
|
|
以上就实现了一个简易的非父子组件之间的通信方式。通过event bus,在一个组件创建时的钩子函数中监听某个事件,而在需要与其进行通信的组件中触发这个函数,同时交换数据。
小站
Vue的非父子组件通信
可以通过vuex和event bus来解决
我们要实现的效果是:
上下分别为foo组件和bar组件,他们之间是非父子关系,分别点击各自的button,另一个组件的count对应增加。
|
|
JavaScript的实现是:
|
|
以上就实现了一个简易的非父子组件之间的通信方式。通过event bus,在一个组件创建时的钩子函数中监听某个事件,而在需要与其进行通信的组件中触发这个函数,同时交换数据。