Vuex讲解
Vuex是什么?
Vuex是一个专为Vue.js应用程序开发的状态管理模式。它采用集中式存储管理应用的所以组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化。
状态管理模式
|
|
这个状态自管理应用包括以下几个部分:
- state:驱动应用的数据源
- view:以声明方式将state映射到视图
- actions:响应在view上的用户输入导致的状态变化。
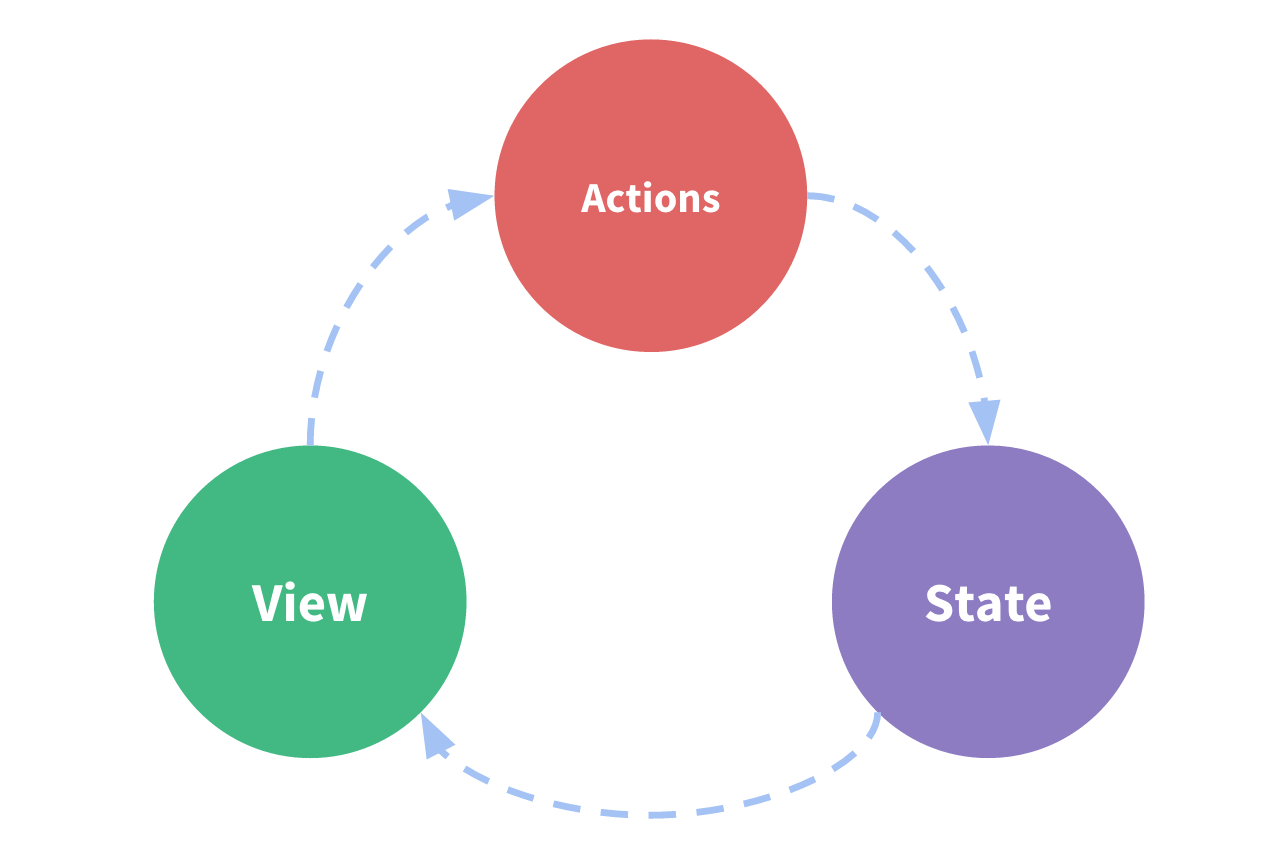
但是,当我们的应用遇到多个组件共享状态时,单向数据流的简洁性很容易被破坏:
- 多个视图依赖于同一状态
- 来自不同视图的行为需要变更同一状态
对于问题一,传参的方法对于多层嵌套的组件将会非常繁琐,并且对于兄弟组件间的状态传递无能为力。对于问题二,我们经常会采用父子组件引用或者通过事件来变更和同步状态的多份拷贝。以上的这些模式非常脆弱,通常会导致代码无法维护。
我们为什么不把组件的共享状态抽取出来,以一个全局单例模式管理呢?在这种模式下,我们的组件树构成了一个巨大的“视图”,不管在树的哪个位置,任何组件都能获取状态或者触发行为。
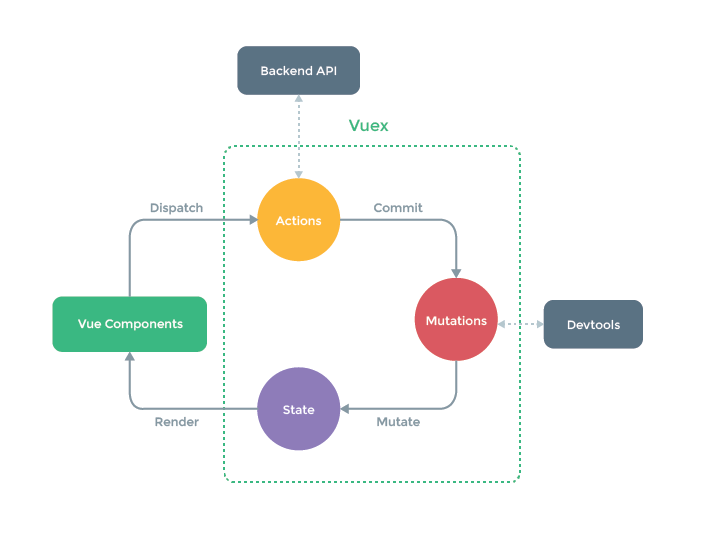
State
单一状态树
Vuex使用 单一状态树,用一个对象就包含了全部的应用层级状态。至此它便作为一个《《唯一数据源》》而存在。这也意味着,每个应用将仅仅包含一个store实例。单一状态树让我们能够直接的定位任意特定的状态片段,在调试的过程中也能够获得当前应用状态的快照。
在Vue组件中获得Vuex状态
Vuex通过store选项,提供了一个机制将状态从根组件注入到每一个子组件中(需调用Vue.use(Vuex)):
通过在根实例中注册store选项,该store实例会注入到根组件的所以子组件中,且子组件能通过this.$store访问到。counter的实现如下:
mapState辅助函数
当一个组件需要获取多个状态的时候,将这些状态都声明为计算属性会有些重复和冗余。可以使用mapState辅助函数帮助我们生成计算属性
Getters
有时候我们需要从store中的state中派生出一些状态,例如对列表进行过滤并计数:
如果有多个组件需要用到此属性,我们要么复制这个函数,或者抽取到一个共享函数然后在多处导入它—无论哪种方式都不是很理想。
Vuex允许我们在store中定义getter(可以认为是store的计算属性)。Getter接受state作为第一个参数:
Getter会暴露为store.getters对象:store.getters.doneTodos
Getters也可以接受其它getters作为第二个参数:
我们可以很容易地在任何组件中使用它:
mapGetters 辅助函数
mapGetters 辅助函数仅仅是将store中的getters映射到局部计算属性:
|
|
如果你想将一个getter属性另取一个名字,使用对象形式:
|
|
Mutations
更改Vuex的store中的状态的唯一方法是提交mutation。Vuex中的mutations非常类似于事件:每个mutation都有一个字符串的事件类型和一个回调函数。这个回调函数就是我们实际进行状态更改的地方,并且它会接受state作为第一个参数:
|
|
不能直接调用一个mutation.handler。这个选项更像是事件注册:“当触发一个类型为increment的mutation时,调用此函数。”要唤醒一个mutation handler,你需要以相应的type调用store.commit方法。store.commit(‘increment’)
提交载荷
你可以向store.commit传入额外的参数,即mutation的载荷:
在大多数情况下,载荷应该是一个对象,这样可以包含多个字段并且记录的mutation会更易读:
|
|
Mutation需遵循Vue的响应规则
既然 Vuex 的 store 中的状态是响应式的,那么当我们变更状态时,监视状态的 Vue 组件也会自动更新。这也意味着 Vuex 中的 mutation 也需要与使用 Vue 一样遵守一些注意事项:
最好提前在你的 store 中初始化好所有所需属性。
当需要在对象上添加新属性时,你应该
使用 Vue.set(obj, ‘newProp’, 123), 或者 -
以新对象替换老对象。例如,利用 stage-3 的对象展开运算符我们可以这样写:
state.obj = { …state.obj, newProp: 123 }
Actions
Action类似于mutation,不同在于:
- Action提交的是mutation,而不是直接变更状态
- Action可以包含任意异步操作
让我们来注册一个简单的action:
Action 函数接受一个与store实例具有相同方法的属性的context对象,因此你可以调用context.commit提交一个mutation,或者通过context.state和context.getters来获取state和getters。
实践中,我们会经常用到ES2015的参数解构来简化代码:
|
|
分发Action
Action通过store.dispatch
方法触发:store.dispatch(‘increment’)
,mutation必须同步执行,但是我们可以在action内部执行异步操作 :
|
|
Actions支持同样的载荷方式和对象方式进行分发:
|
|
在组件中分发Action
你在组件中使用this.$store.dispatch(‘xxx’)
分发action,或者使用mapActions
辅助函数将组件的methods映射为store.dispatch
调用(需要先在根节点注入store):
|
|
组合Actions
Action通常是异步的,那么如何知道action什么时候结束呢?更重要的是,我们如何才能组合多个action,以处理更加复杂的异步流程?
首先,你需要明白store.dispatch
可以处理被触发的action的回调函数返回的Promise,并且store.dispatch仍然返回Promise:
现在你可以:
在另外一个action中也可以:
Modules
由于使用单一状态树,应用的所以状态会集中到一个比较大的对象。当应用变得非常复杂时,store对象就有可能变得相对臃肿。
为了解决以上问题,Vuex允许我们将store分割成模块,每个模块拥有自己的state,mutation,action,getter,甚至是嵌套子模块—从上至下进行同样方式分割:
|
|
模块的局部状态
对于模块内部的mutation和getter,接受的第一个参数是模块的局部状态对象。
同样,对于模块内部的action,局部状态通过context.state
暴露出来,根节点状态则为context.rootState
:
对于模块内部的getter,根节点状态会作为第三个参数暴露出来:
命名空间
默认情况下,模块内部的 action、mutation 和 getter 是注册在全局命名空间的——这样使得多个模块能够对同一 mutation 或 action 作出响应。如果希望你的模块更加自包含或提高可重用性,你可以通过添加 namespaced: true 的方式使其成为命名空间模块。当模块被注册后,它的所有 getter、action 及 mutation 都会自动根据模块注册的路径调整命名。例如:
|
|
启用了命名空间的 getter 和 action 会收到局部化的 getter,dispatch 和 commit。换言之,你在使用模块内容(module assets)时不需要在同一模块内额外添加空间名前缀。更改 namespaced 属性后不需要修改模块内的代码。